How to add extra custom fields in to the default Customer Registration page in Magento 2
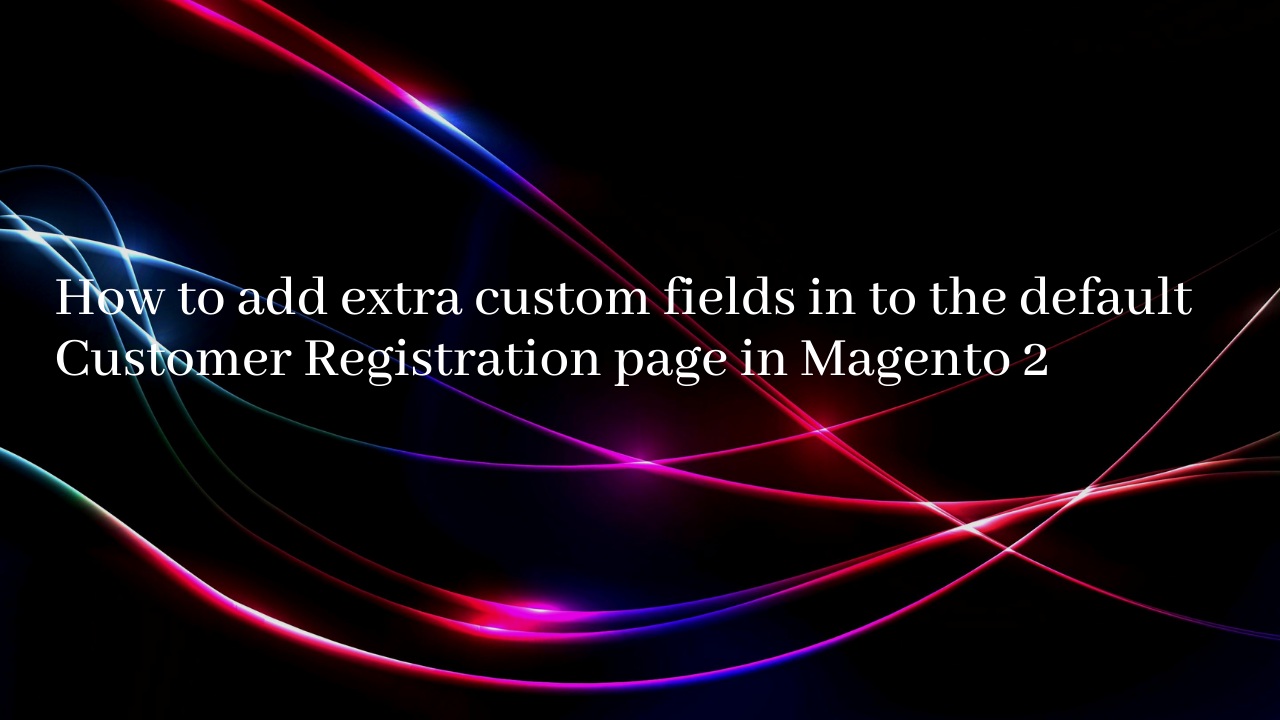
1.Create a Module in app/code(Example: Say_Hello).
Then create a registration.php in app/code/Say/Hello/registration.php
<?php
use Magento\Framework\Component\ComponentRegistrar;
ComponentRegistrar::register(
ComponentRegistrar::MODULE,
'Say_Hello',
__DIR__
);
2.Create a composer.json file for your module.
{
"name": "say/module-hello",
"description": "Online accessories store",
"type": "magento2-module",
"minimum-stability": "stable",
"require": {
"magento/module-catalog": "^103.0.*",
"mageworx/module-hello": "^1.5.*"
},
"autoload": {
"files": [
"registration.php"
],
"psr-4": {
"Say\\Hello\\": ""
}
}
}
3.Create a module.xml in app/code/Say/Hello/etc/module.xml.
<?xml version="1.0" ?>
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Say_Hello" setup_version="1.0.0">
<sequence>
<module name="Magento_Customer"/>
</sequence>
</module>
</config>
4.Create a InstallData.php in app/code/Say/Hello/Setup/InstallData.php(under Setup folder).For creating additional customer attribute.
<?php
namespace Say\Hello\Setup;
use Magento\Customer\Setup\CustomerSetupFactory;
use Magento\Customer\Model\Customer;
use Magento\Eav\Model\Entity\Attribute\Set as AttributeSet;
use Magento\Eav\Model\Entity\Attribute\SetFactory as AttributeSetFactory;
use Magento\Framework\Setup\InstallDataInterface;
use Magento\Framework\Setup\ModuleContextInterface;
use Magento\Framework\Setup\ModuleDataSetupInterface;
class InstallData implements InstallDataInterface
{
/**
* @var CustomerSetupFactory
*/
protected $customerSetupFactory;
/**
* @var AttributeSetFactory
*/
private $attributeSetFactory;
/**
* @param CustomerSetupFactory $customerSetupFactory
* @param AttributeSetFactory $attributeSetFactory
*/
public function __construct(
CustomerSetupFactory $customerSetupFactory,
AttributeSetFactory $attributeSetFactory
) {
$this->customerSetupFactory = $customerSetupFactory;
$this->attributeSetFactory = $attributeSetFactory;
}
public function install(ModuleDataSetupInterface $setup, ModuleContextInterface $context)
{
/*customersetupfactory instead of eavsetupfactory */
$customerSetup = $this->customerSetupFactory->create(['setup' => $setup]);
$customerEntity = $customerSetup->getEavConfig()->getEntityType('customer');
$attributeSetId = $customerEntity->getDefaultAttributeSetId();
/** @var $attributeSet AttributeSet */
$attributeSet = $this->attributeSetFactory->create();
$attributeGroupId = $attributeSet->getDefaultGroupId($attributeSetId);
/* create customer phone number attribute */
$customerSetup->addAttribute(Customer::ENTITY,'mobile_no', [
'type' => 'varchar',
'label' => 'Mobile No',
'input' => 'text',
'required' => false,
'visible' => true,
'user_defined' => true,
'position' => 999,
'sort_order' => 999,
'system' => 0,
'is_used_in_grid' => 1,
'is_visible_in_grid' => 1,
'is_filterable_in_grid' => 1,
'is_searchable_in_grid' => 1,
]
);
$sampleAttribute = $customerSetup->getEavConfig()->getAttribute(Customer::ENTITY, 'mobile_no')
->addData(
[
'attribute_set_id' => $attributeSetId,
'attribute_group_id' => $attributeGroupId,
'used_in_forms' => ['adminhtml_customer','customer_account_edit','customer_account_create'],
]
$sampleAttribute->save();
}
}
5.Create a view file additional.phtml in app/code/Say/Hello/view/frontend/templates/additional.phtml to show additional field on customer registration form.
<div class="field mobile number required">
<label class="label" for="mobileno">
<span><?= $block->escapeHtml(__('Mobile No')) ?></span>
</label>
<div class="control">
<input type="text" name="mobile_no" id="mobile_no" value="" title="<?= $block->escapeHtmlAttr(__('Mobile No')) ?>" class="input-text" data-validate="{required:true}">
</div>
</div>
6.Create layout file named customer_account_create.xml in app/code/Say/Hello/view/frontend/layout/customer_account_create.xml.
<?xml version="1.0"?>
<page xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" layout="1column" xsi:noNamespaceSchemaLocation="urn:magento:framework:View/Layout/etc/page_configuration.xsd">
<body>
<referenceContainer name="form.additional.info">
<block class="Magento\Framework\View\Element\Template" name="form_additional_info_customer" template="Say_Hello::additional.phtml"/>
</referenceContainer>
</body>
</page>
7.At last run the following command.
php bin/magento setup:upgrade
php bin/magento setup:static-content:deploy -f
php bin/magento cache:flush
The mobile field will show like this.
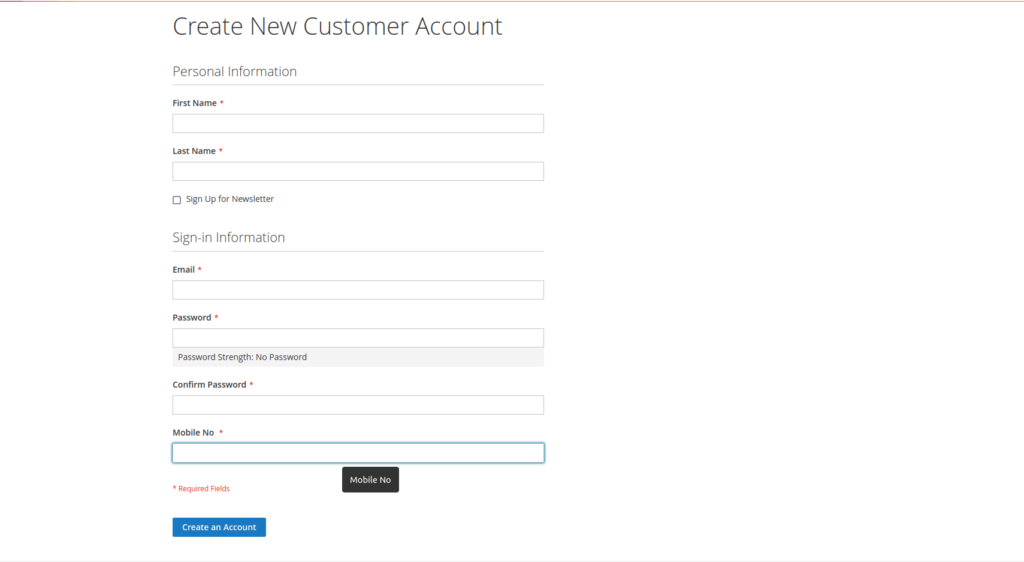